How to Can Redemption Machines Read Barcodes
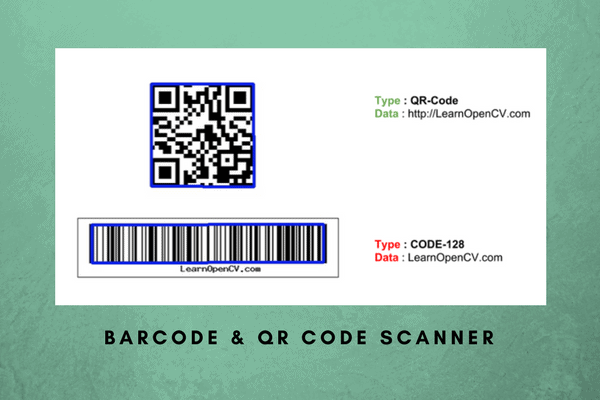
In this post, we volition share C++ and Python code for writing a barcode and QR code scanner using a library chosen ZBar and OpenCV. The Python lawmaking works in both Python 2 and Python iii.
If you lot have never seen a barcode or a QR lawmaking, please send me the accost of your cave and so I can send you lot a sample past mail. Jokes aside, barcodes and QR codes are everywhere. In fact, I have a QR code on the back of my business organization card besides! Pretty cool, huh?
How to detect and decode barcodes and QR codes in an image?
Step ane : Install ZBar
The best library for detecting and decoding barcodes and QR codes of different types is called ZBar. Before nosotros begin, you demand to download and install ZBar by following the instructions here.
macOS users tin can simply install using Homebrew
Ubuntu users tin install using
sudo apt-go install libzbar-dev libzbar0
Step ii : Install pyzbar (for Python users but)
The official version of ZBar does non support Python 3. Then nosotros recommend using pyzbar which supports both ython ii and Python iii. If you only want to piece of work with python 2, you can install zbar and skip installing pyzbar.
Install ZBar
# Note: Official zbar version does not support Python 3 pip install zbar
Install pyzbar
Download Code To easily follow along this tutorial, please download lawmaking by clicking on the button below. It's FREE!
Step 3: Understanding the construction of a barcode / QR code
A barcode / QR lawmaking object returned by ZBar has iii fields
- Blazon: If the symbol detected by ZBar is a QR code, the type is QR-Code. If it is barcode, the type is one of the several kinds of barcodes ZBar is able to read. In our case, we have used a barcode of type Lawmaking-128
- Data: This is the data embedded inside the barcode / QR lawmaking. This data is usually alphanumeric, but other types ( numeric, byte/binary etc. ) are also valid.
- Location: This is a collection of points that locate the code. For QR codes, it is a list of four points respective to the four corners of the QR code quad. For barcodes, location is a drove of points that mark the start and cease of word boundaries in the barcode. The location points are plotted for a few different kinds of symbols below.
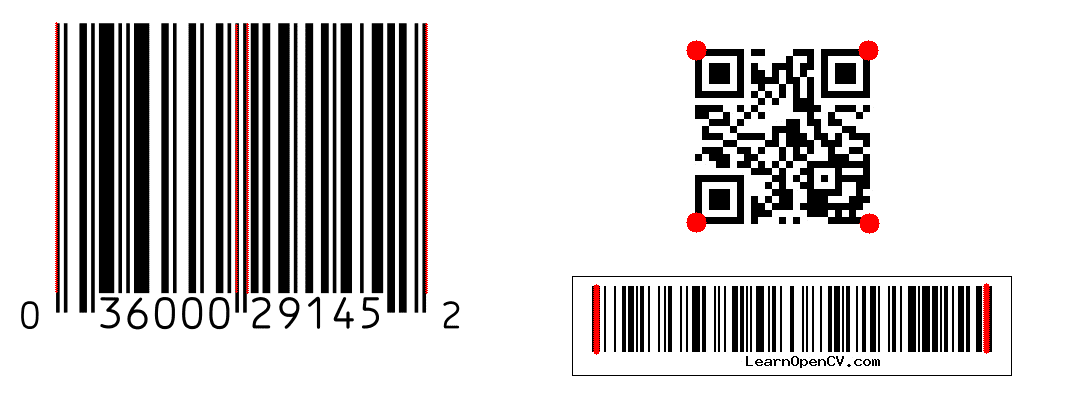
Step 3a : C++ code for scanning barcode and QR code using ZBar + OpenCV
Nosotros first define a struture to concord the information about a barcode or QR code detected in an image.
typedef struct { string type; cord information; vector <Indicate> location; } decodedObject;
The type, data, and location fields are explained in the previous section.
Download Code To easily follow forth this tutorial, please download code by clicking on the push button beneath. It'due south Gratis!
Let's wait at the decode function that takes in an image and returns all barcodes and QR codes found.
// Find and decode barcodes and QR codes void decode(Mat &im, vector<decodedObject>&decodedObjects) { // Create zbar scanner ImageScanner scanner; // Configure scanner scanner.set_config(ZBAR_NONE, ZBAR_CFG_ENABLE, 1); // Convert image to grayscale Mat imGray; cvtColor(im, imGray,CV_BGR2GRAY); // Wrap prototype data in a zbar image Image image(im.cols, im.rows, "Y800", (uchar *)imGray.data, im.cols * im.rows); // Scan the epitome for barcodes and QRCodes int n = scanner.scan(epitome); // Print results for(Paradigm::SymbolIterator symbol = image.symbol_begin(); symbol != image.symbol_end(); ++symbol) { decodedObject obj; obj.type = symbol->get_type_name(); obj.data = symbol->get_data(); // Print type and data cout << "Type : " << obj.type << endl; cout << "Data : " << obj.data << endl << endl; // Obtain location for(int i = 0; i< symbol->get_location_size(); i++) { obj.location.push_back(Point(symbol->get_location_x(i),symbol->get_location_y(i))); } decodedObjects.push_back(obj); } }
First, in lines 5-9 we create an instance of a ZBar ImageScanner and configure it to detect all kinds of barcodes and QR codes. If you lot want simply a specific kind of symbol to be detected, yous need to alter ZBAR_NONE to a unlike type listed here. We and then convert the image to grayscale ( lines xi-13). Nosotros then catechumen the grayscale image to a ZBar compatible format in line 16 . Finally, nosotros scan the prototype for symbols (line nineteen). Finally, we iterate over the symbols and excerpt the type, data, and location information and button it in the vector of detected objects (lines 21-twoscore).
Next, we volition explain the code for displaying all the symbols. The code below takes in the input paradigm and a vector of decoded symbols from the previous step. If the points form a quad ( e.grand. in a QR code ), we simply draw the quad ( line 14 ). If the location is not a quad, nosotros draw the outer boundary of all the points ( likewise called the convex hull ) of all the points. This is washed using OpenCV function called convexHull shown in line 12.
// Display barcode and QR code location void display(Mat &im, vector<decodedObject>&decodedObjects) { // Loop over all decoded objects for(int i = 0; i < decodedObjects.size(); i++) { vector<Point> points = decodedObjects[i].location; vector<Point> hull; // If the points do not form a quad, find convex hull if(points.size() > 4) convexHull(points, hull); else hull = points; // Number of points in the convex hull int n = hull.size(); for(int j = 0; j < northward; j++) { line(im, hull[j], hull[ (j+1) % n], Scalar(255,0,0), 3); } } // Brandish results imshow("Results", im); waitKey(0); }
Finally, we have the main function shared below that simply reads an image, decodes the symbols using the decode part described above and displays the location using the brandish role described above.
int principal(int argc, char* argv[]) { // Read image Mat im = imread("zbar-test.jpg"); // Variable for decoded objects vector<decodedObject> decodedObjects; // Detect and decode barcodes and QR codes decode(im, decodedObjects); // Brandish location display(im, decodedObjects); return EXIT_SUCCESS; }
Step 3b : Python code for scanning barcode and QR lawmaking using ZBar + OpenCV
For Python, we use pyzbar, which has a unproblematic decode function to locate and decode all symbols in the image. The decode role in lines 6-xv but warps pyzbar's decode part and loops over the located barcodes and QR codes and prints the data.
The decoded symbols from the previous step are passed on to the display function (lines nineteen-41). If the points form a quad ( e.k. in a QR code ), we but draw the quad ( line 30 ). If the location is non a quad, we draw the outer boundary of all the points ( as well chosen the convex hull ) of all the points. This is done using OpenCV part called cv2.convexHull shown in line 27.
Finally, the chief function simply reads an image, decodes it and displays the results.
from __future__ import print_function import pyzbar.pyzbar equally pyzbar import numpy equally np import cv2 def decode(im) : # Discover barcodes and QR codes decodedObjects = pyzbar.decode(im) # Print results for obj in decodedObjects: print('Type : ', obj.type) impress('Information : ', obj.data,'\n') return decodedObjects # Display barcode and QR code location def display(im, decodedObjects): # Loop over all decoded objects for decodedObject in decodedObjects: points = decodedObject.polygon # If the points do not form a quad, detect convex hull if len(points) > four : hull = cv2.convexHull(np.array([point for point in points], dtype=np.float32)) hull = listing(map(tuple, np.squeeze(hull))) else : hull = points; # Number of points in the convex hull north = len(hull) # Describe the convext hull for j in range(0,n): cv2.line(im, hull[j], hull[ (j+1) % due north], (255,0,0), 3) # Display results cv2.imshow("Results", im); cv2.waitKey(0); # Primary if __name__ == '__main__': # Read image im = cv2.imread('zbar-exam.jpg') decodedObjects = decode(im) display(im, decodedObjects)
Some Results
Subscribe & Download Code
If you liked this article and would like to download code (C++ and Python) and case images used in this mail, please click here. Alternately, sign up to receive a free Estimator Vision Resource Guide. In our newsletter, we share OpenCV tutorials and examples written in C++/Python, and Estimator Vision and Machine Learning algorithms and news.
Source: https://learnopencv.com/barcode-and-qr-code-scanner-using-zbar-and-opencv/
0 Response to "How to Can Redemption Machines Read Barcodes"
Post a Comment